JavaScript Map for Objects
JavaScript Map for objects is a data structure that allows the storage of key-value pairs where both key and value can be of any type, including objects. In fact, the Map data structure is particularly useful for working with complex data types, like objects, because it supports key-based access and iteration.
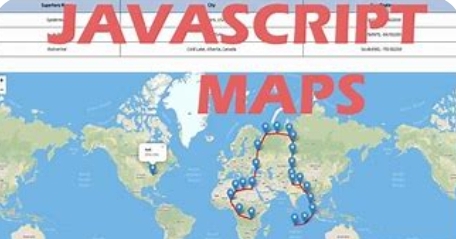
Using JavaScript Map for objects involves creating a new Map instance and using object references as keys. Here’s an example of how to create and use a JavaScript Map for objects:
“`
// Create a Map for object data
const dataMap = new Map();
// Define an object to store in the Map
const objData = {
firstName: ‘John’,
lastName: ‘Doe’,
age: 30
};
// Use the object reference as the Map key
dataMap.set(objData, 12345);
// Get the value associated with the object key
const value = dataMap.get(objData);
console.log(value); // Output: 12345
“`
In this example, we create a new Map instance called `dataMap`. We then define an object `objData` and use it as the key to set a value of `12345` in the Map. We retrieve the value from the Map using the same object reference as the key, and output it to the console.
JavaScript Map for objects also provides some additional methods, like `has()` and `delete()`, for working with key-value pairs and objects. By using JavaScript Maps for objects, we can simplify our code and improve its readability and complexity.
YOU CAN ALSO READ : JAVASCRIPT IN ARRAY: THE ART OF DIGITAL EXPRESSION
Can you use a map on JavaScript Map for objects?
Yes, you can use a Map object in JavaScript to store and retrieve key-value pairs. A Map object is similar to a regular object, but provides additional functionality such as allowing any type of object to be used as a key and preserving the order of insertion. Here is an example of using a Map object in JavaScript:
“`
// Create a new Map object
let myMap = new Map();
// Add a key-value pair to the Map
myMap.set(‘key’, ‘value’);
// Retrieve a value from the Map using the key
let value = myMap.get(‘key’);
// Check if the Map contains a key
let containsKey = myMap.has(‘key’);
“`
In this example, the `myMap` object is created using the `Map()` constructor, and a key-value pair is added using the `set()` method. The `get()` method is used to retrieve the value associated with a key, and the `has()` method is used to check if a key exists in the Map object.
javascript map object examples
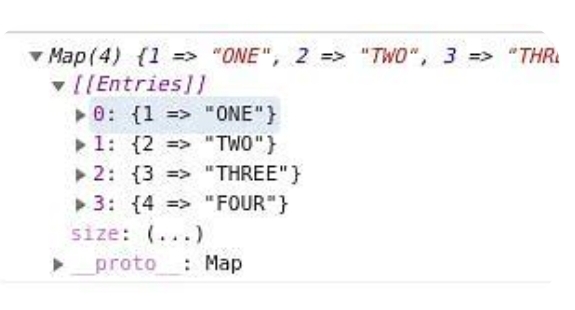
1. Example of mapping an array of numbers and squaring each number:
“`
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(num => num * num);
console.log(squaredNumbers); // [1, 4, 9, 16, 25]
“`
2. Example of mapping an array of objects and retrieving a specific property:
“`
const users = [
{ name: “John”, age: 25 },
{ name: “Mary”, age: 32 },
{ name: “Michael”, age: 19 }
];
const userNames = users.map(user => user.name);
console.log(userNames); // [“John”, “Mary”, “Michael”]
“`
3. Example of mapping an array of strings and capitalizing each string:
“`
const fruits = [“apple”, “banana”, “orange”];
const capitalizedFruits = fruits.map(fruit => fruit.toUpperCase());
console.log(capitalizedFruits); // [“APPLE”, “BANANA”, “ORANGE”]
“`
4. Example of mapping an array of numbers and adding 10 to each number:
“`
const numbers = [1, 2, 3, 4, 5];
const addedNumbers = numbers.map(num => num + 10);
console.log(addedNumbers); // [11, 12, 13, 14, 15]
“`
5. Example of mapping an array of objects and adding a new property:
“`
const users = [
{ name: “John”, age: 25 },
{ name: “Mary”, age: 32 },
{ name: “Michael”, age: 19 }
];
const newUserList = users.map(user => {
return { …user, isLoggedIn: true };
});
console.log(newUserList);
/* Output:
[
{ name: “John”, age: 25, isLoggedIn: true },
{ name: “Mary”, age: 32, isLoggedIn: true },
{ name: “Michael”, age: 19, isLoggedIn: true }
]
*/
YOU CAN ALSO READ : THE USE OF BLAZOR VS JAVASCRIPT IN WEB DEVELOPMENT
Can we use map in javascript?
Yes, we can use the `Map` object in JavaScript. The `Map` object is a collection of key-value pairs that allows you to store and retrieve data with a custom key. The keys in a `Map` object can be of any type, including objects and functions. Here is an example of using `Map`:
“`
const myMap = new Map();
myMap.set(‘key1’, ‘value1’);
myMap.set(2, ‘value2’);
myMap.set({name: ‘John’}, ‘value3’);
console.log(myMap.get(‘key1’));
// Output: value1
console.log(myMap.get(2));
// Output: value2
console.log(myMap.get({name: ‘John’}));
// Output: value3
“`
In the example, we create a new `Map` object and add key-value pairs to it using the `set()` method. We then retrieve the values of the keys using the `get()` method.
We can also use `Map` methods such as `has()`, `delete()` and `clear()` to check and modify the `Map`. Here’s an example:
“`
const myMap = new Map();
myMap.set(‘key1’, ‘value1’);
myMap.set(‘key2’, ‘value2’);
console.log(myMap.has(‘key1’));
// Output: true
myMap.delete(‘key2’);
console.log(myMap.has(‘key2’));
// Output: false
myMap.clear();
console.log(myMap.size);
// Output: 0
“`
In this example, we first check if the `Map` has a key with the value `”key1″` using the `has()` method. Then, we delete a key-value pair from the `Map` using the `delete()` method. Finally, we clear the `Map` using the `clear()` method, which removes all key-value pairs from the `Map`.
can you use map on an object javascript
No, you cannot use the `map` method on an object in JavaScript because `map` only works on arrays. If you need to transform the properties of an object, you can use a `for…in` loop to iterate over the properties and apply the transformation manually. Alternatively, you can convert the object into an array of key-value pairs using the `Object.entries` method, then use `map` on the resulting array.
javascript map list of objects
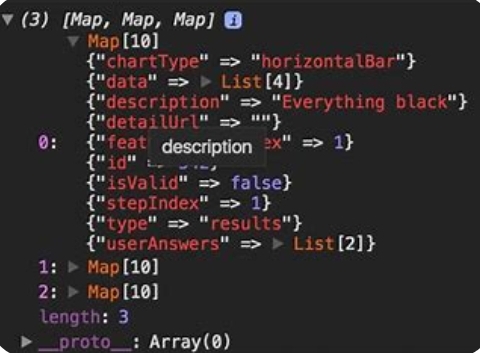
In JavaScript Map for objects , map is a higher-order function that allows you to transform the items in an array. When it is used on a list of objects, it can be used to manipulate the object properties, add or remove elements from objects, or create new objects based on the existing objects in the list.
Here’s an example of how to use map on a list of objects:
“`
const employees = [
{ name: ‘John’, age: 32, department: ‘IT’ },
{ name: ‘Jane’, age: 27, department: ‘HR’ },
{ name: ‘Bob’, age: 45, department: ‘Marketing’ }
];
const employeeNames = employees.map((employee) => {
return employee.name;
});
console.log(employeeNames); // Output: [‘John’, ‘Jane’, ‘Bob’]
“`
In the example above, we have a list of employee objects. We use map to create a new array `employeeNames` that contains only the names of the employees. We pass a callback function to map that takes in each object in the array (`employee`) and returns the name property of that object.
We can also use map to create new objects based on the existing objects in the list. For example:
“`
const employeeDetails = employees.map((employee) => {
return { name: employee.name, department: employee.department };
});
console.log(employeeDetails);
// Output: [{name: ‘John’, department: ‘IT’}, {name: ‘Jane’, department: ‘HR’}, {name: ‘Bob’, department: ‘Marketing’}]
“`
In this example, we create a new array `employeeDetails` containing only the name and department properties of each employee object. We pass a callback function to map that takes in each object in the array (`employee`) and returns a new object with only the name and department properties.
Overall, using map on a list of objects allows you to transform and manipulate the objects in the array in many different ways based on your needs.
how to use javascript .map() for array of objects
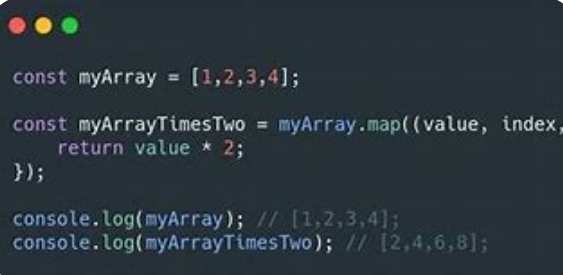
The JavaScript Map for objects ()` method in JavaScript allows you to iterate over an array and modify each element in it. When applied to an array of objects, `.map()` allows you to access and manipulate the properties of each object.
Here’s how you can use `.map()` on an array of objects:
1. Create an array of objects:
“`javascript
const originalArray = [
{ id: 1, name: “John” },
{ id: 2, name: “Jane” },
{ id: 3, name: “James” }
];
“`
2. Call the `.map()` method on the array and pass in a callback function:
“`javascript
const modifiedArray = originalArray.map((object) => {
// Code to modify each object goes here
});
“`
3. Inside the callback function, you can access the current object as a parameter and modify its properties as needed. The modified object will be returned to the new array created by `.map()`.
“`javascript
const modifiedArray = originalArray.map((object) => {
// Access and modify properties of each object
return {
…object,
newName: object.name.toUpperCase(),
age: calculateAge(object.birthYear) // You can also call external functions
};
});
“`
In this example, we added a new property `newName` to each object, which is the uppercase version of the original `name` property. We also added an `age` property calculated using an external function.
4. Finally, after calling `.map()`, `modifiedArray` will contain the transformed objects:
“`javascript
console.log(modifiedArray);
“`
Output:
“`javascript
[
{ id: 1, name: “John”, newName: “JOHN”, age: 30 },
{ id: 2, name: “Jane”, newName: “JANE”, age: 28 },
{ id: 3, name: “James”, newName: “JAMES”, age: 25 }
]
“`
Note that `.map()` does not modify the original array; it creates a new array with the modified objects. You can assign this new array to a variable (`modifiedArray` in this example) to use it elsewhere in your code.
That’s how you can use `.map()` to loop through an array of objects and modify them.
YOU CAN ALSO READ : THE ULTIMATE CHECKLIST FOR BLAZOR WEBASSEMBLY
can you use map on an object javascript
Yes, it is possible to use the `map` method on an object in JavaScript. However, unlike arrays, objects do not have a built-in `map` method. To use `map` on an object, you can:
1. Convert the object into an array of entries using the `Object.entries` method, which returns an array of key-value pairs (each pair represented as an array).
2. Use `map` on the resulting array of entries to transform the values.
3. Convert the transformed array back into an object using the `Object.fromEntries` method, which takes an array of key-value pairs and returns a new object.
Here is an example code snippet to illustrate how this works:
“`
const originalObj = {a: 1, b: 2, c: 3};
const newObj = Object.fromEntries(Object.entries(originalObj).map(([key, value]) => {
return [key, value * 2];
}));
console.log(newObj); // {a: 2, b: 4, c: 6}
“`
In this example, we start with an object `originalObj` with three key-value pairs. We use `Object.entries` to convert it into an array of entries. We then use `map` to transform each value by multiplying it by 2. Finally, we use `Object.fromEntries` to convert the transformed array back into an object, which we store in `newObj`. The resulting `newObj` has the same keys as the original object, but with multiplied values.
javascript map object destructuring
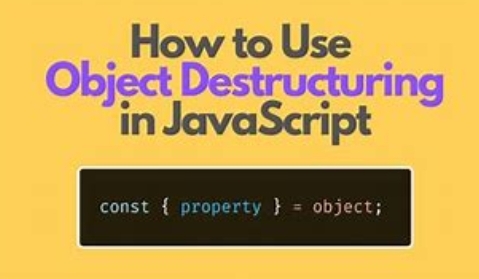
JavaScript map object destructuring is a way to extract and assign values from an object to variables by using the curly braces ({}) around the keys that we want to extract. In the case of map objects, we use the map’s `entries()` method to get an array of key-value pairs, which we can then destructure using the curly braces.
For example:
“`js
const myMap = new Map();
myMap.set(‘firstName’, ‘John’);
myMap.set(‘lastName’, ‘Doe’);
myMap.set(‘age’, 30);
// Destructuring the map object
const { firstName, lastName } = Object.fromEntries(myMap.entries());
console.log(firstName); // “John”
console.log(lastName); // “Doe”
“`
Here, we create a `myMap` object with key-value pairs for `firstName`, `lastName`, and `age`. We then use the `entries()` method to get an array of the key-value pairs, which we pass to the `Object.fromEntries()` method to convert back to an object. Finally, we destructure the object to extract the values for `firstName` and `lastName`.
javascript map object delete key
To delete a key from a JavaScript map object, you can use the `delete` keyword in combination with the map object and the key you want to remove:
“`javascript
let myMap = new Map();
myMap.set(“key1”, “value1”);
myMap.set(“key2”, “value2”);
myMap.set(“key3”, “value3”);
myMap.delete(“key2”); // delete key2
console.log(myMap); // output: Map(2) { ‘key1’ => ‘value1’, ‘key3’ => ‘value3’ }
“`
In the example above, we created a new map object `myMap` and added three key-value pairs. To delete `key2`, we used the `delete` keyword followed by the key we wanted to remove. Finally, we printed the remaining keys and their values to the console using `console.log(myMap)`.
javascript map object difference
In JavaScript Map for objects , a map object is a collection of key-value pairs. The main difference between a map object and other objects in JavaScript is that the keys in a map object can be of any data type, whereas the keys in other objects are always strings. Additionally, map objects are iterable, which means you can loop through them using methods like forEach() or for…of loops.
Another difference is that maps preserve the order in which items were added, while other objects do not. This means that when you loop through a map object, you will get the items in the order in which they were added.
Lastly, JavaScript Map for objects have built-in methods to get the size of the map (the number of key-value pairs), to add and remove items, and to check if a key exists in the map. These methods make it easy to work with maps and manipulate them as needed.
javascript map object display
The JavaScript Map for objects is used to store key-value pairs and allows us to store any type of data corresponding to any type of key. In order to display the contents of a Map object, we can use either the for…of loop or the forEach() method.
Using for…of loop:
“`
let myMap = new Map();
myMap.set(“key1”, “value1”);
myMap.set(“key2”, “value2”);
for (let [key, value] of myMap) {
console.log(key + ” = ” + value);
}
“`
Output:
“`
key1 = value1
key2 = value2
“`
Using forEach() method:
“`
let myMap = new Map();
myMap.set(“key1”, “value1”);
myMap.set(“key2”, “value2”);
myMap.forEach(function(value, key) {
console.log(key + ” = ” + value);
});
“`
Output:
“`
key1 = value1
key2 = value2
“`
In both methods, we are iterating through the Map object and accessing the key and value pairs using destructuring assignment. We can then display the key-value pairs using console.log() or any other appropriate method.
javascript map object equality
In JavaScript Map for objects, two object literals or objects created using the constructor function are considered equal only if they refer to the same object in memory.
However, when comparing objects in an array or using a Map object, two objects with identical key-value pairs are not considered equal unless they refer to the same object.
For example:
“`
const obj1 = {name: ‘John’, age: 35};
const obj2 = {name: ‘John’, age: 35};
const obj3 = obj1;
console.log(obj1 === obj2); //false
console.log(obj1 === obj3); //true
const map = new Map();
map.set(obj1, ‘value1’);
console.log(map.has(obj1)); //true
console.log(map.has(obj2)); //false
“`
In the above example, `obj1` and `obj2` have identical key-value pairs, but they are not considered equal because they refer to different objects in memory. On the other hand, `obj1` and `obj3` refer to the same object in memory, so they are considered equal.
Similarly, when using a Map object, `obj1` is used as a key, and the value `value1` is associated with it. When checking for the existence of `obj1` using `map.has()`, the function returns true because `obj1` is stored as a key in the map, but `obj2` is not considered equal to `obj1`, so `map.has(obj2)` returns false.
javascript map object es5
In ES5 (ECMAScript 5), the Map object was introduced to provide an efficient means to store key-value pairs and access them quickly. The Map object is a collection of key-value pairs where each key maps to a value, and keys can be of any type, including objects and functions.
The following are the key features of the Map object in ES5:
1. The JavaScript Map for objects is an ordered collection of key-value pairs where the order of the keys is maintained.
2. The JavaScript Map for objects provides a way to store and access key-value pairs in an efficient manner.
3. The JavaScript Map for objects can use any data type as a key or value, including objects and functions.
4. The JavaScript Map for objects provides convenient methods to retrieve the keys, values, and entries of the map.
5. The JavaScript Map for objects has a size property that returns the number of key-value pairs in the map.
6. The JavaScript Map for objects has a set() method to add new key-value pairs to the map.
7. The JavaScript Map for objects has a get() method to retrieve a value associated with a particular key.
8. The JavaScript Map for objects has a delete() method to remove the key-value pair associated with a particular key.
9. The JavaScript Map for objects has a clear() method to remove all key-value pairs from the map.
Overall, the Map object in ES5 provides a better way to manage key-value pairs and is more efficient than using traditional object literals in JavaScript.
YOU CAN ALSO READ : THE UNIVERSAL BENDING MAGIC OF REPLACE ALL WITH JAVASCRIPT.
javascript map equivalent for object
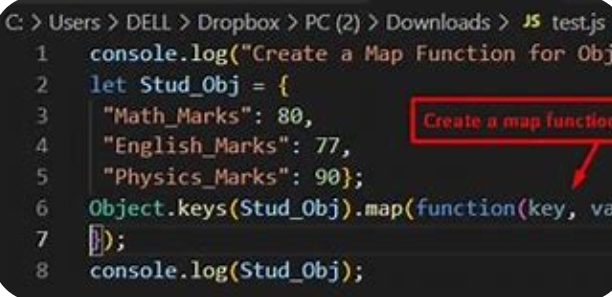
The equivalent of `Array.map()` method for objects is `Object.entries()` method.
`Object.entries()` method returns an array of a given object’s own enumerable string-keyed property [key, value] pairs, in the same order as that provided by a for…in loop.
Example:
“`
const obj = { a: 1, b: 2, c: 3 };
const newObj = Object.fromEntries(
Object.entries(obj).map(([ key, val ]) => [ key, val * 2 ])
);
console.log(newObj); // { a: 2, b: 4, c: 6 }
“`
In the above example, `Object.entries()` converts the object into an array of [key, value] pairs. Then, the `map()` method is used to iterate over the array and double the values of each property. Finally, the `Object.fromEntries()` method is used to convert the array back into an object.
javascript map return different object
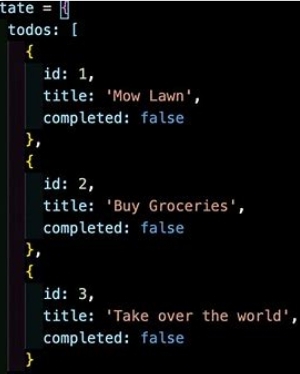
Yes, when using the map method in JavaScript, you can return a different object than the original object that was mapped over. This is because the map method creates a new array with the same number of elements as the original array, but with each element transformed according to the function provided.
For example, consider an array of numbers:
“`
const numbers = [1, 2, 3, 4, 5];
“`
If we map over this array and return a new array where each number is doubled:
“`
const doubledNumbers = numbers.map(num => num * 2);
“`
The resulting array `doubledNumbers` will have the same length as `numbers`, but each element will be twice the value of the corresponding element in `numbers`. In this case, we are returning a different object (a new array) than the original object that was mapped over (`numbers`).