Replace all with javascript: JavaScript stands as a pivotal programming language that empowers dynamic and interactive web pages. One particularly handy function within the JavaScript arsenal is the “replace all with javascript” method. This method not only streamlines coding processes but also enhances the overall user experience.
Replace all with javascript : The “replace all with javascript” function, as the name suggests, is designed to replace all occurrences of a specified substring or pattern within a given string. This process eliminates the need to manually iterate through the string and replace each occurrence individually. Instead, developers can harness the power of this function to achieve the same result with fewer lines of code and enhanced efficiency.

Replace all with javascript : One of the common scenarios where the “replace all with javascript” function proves its worth is in form validation. Consider a user input field where certain characters, such as spaces or special symbols, need to be removed or replaced. Instead of implementing a cumbersome loop to address each instance, developers can employ the “replace all” method to swiftly replace all occurrences, ensuring clean and consistent data.
Replace all with javascript : The “replace all with javascript” function isn’t confined to simple text replacements. It can also be extended to manipulate more complex patterns using regular expressions. This versatility empowers developers to tackle a wide array of tasks without unnecessary complexity.
There are moments which a developer will need to replace all array elements with new data. While this looks like a simple task, it can be complex for beginners who are yet to get familiar with the syntax and how JavaScript arrays function. In this article you will learn how to change all JavaScript arrays.
YOU CAN ALSO READ : JAVASCRIPT IN ARRAY: THE ART OF DIGITAL EXPRESSION
Replace all with javascript apostrophe
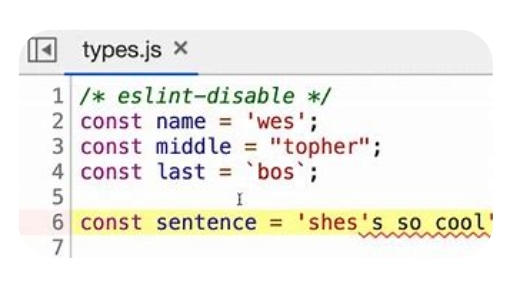
To replace all apostrophes (‘) in a string with another character or string using JavaScript, you can use the `.replace()` method with a regular expression.
For example, to replace all apostrophes with a dash (-) in a given string variable `myString`, you can use the following code:
“`
myString = myString.replace(/’/g, ‘-‘);
“`
Here, the regular expression `/’/g` matches all occurrences of apostrophes in the string and the `g` flag indicates to replace all matches, not just the first one. The replacement string `-` is used to replace each matched apostrophe.
You can replace the dash (-) with any other character or string as desired.
Replace all javascript array
JavaScript arrays are essential tools for managing and manipulating different sets of data in web development. We have written an article on JavaScript arrays so do well to read it up for a detailed understanding of what a JavaScript array is. JavaScript arrays are useful for organizing big datasets and can also be used for a wider purpose for example; JavaScript arrays can be used to create dynamic menus which handle complex forms.
There are moments which a developer will need to replace all array elements with new data. While this looks like a simple task, it can be complex for beginners who are yet to get familiar with the syntax and how JavaScript arrays function. In this article you will learn how to change all JavaScript arrays.
YOU CAN ALSO READ: THE USE OF BLAZOR VS JAVASCRIPT IN WEB DEVELOPMENT
javascript replace all characters with asterisk
If you to replace all characters with asterisks in a JavaScript string, you can use the replace() function with a regular expression that matches any character. Here’s an example:
“`javascript
let originalString = “This is a sensitive message!”;
let maskedString = originalString.replace(/./g, “*”);
console.log(maskedString); // Output: “**********************”
“`
In this code, the regular expression `/./g` matches any character in the original string, and the “g” flag makes it replace all occurrences of the pattern. The second argument to the replace() function is the replacement string, which is just a single asterisk in this case.
Note that this code will replace all characters in the string, including spaces and punctuation. If you want to exclude certain characters from being masked, you can modify the regular expression to match only specific characters.
javascript replace all characters with another
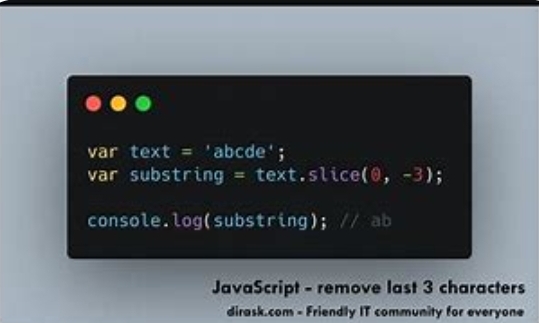
You can use the `replaceAll()` method to replace all occurrences of a character with another character in a string:
“`
let str = “hello world”;
str = str.replaceAll(“l”, “z”);
console.log(str); // “hezzo worzd”
“`
In the above example, all occurrences of the letter “l” in the string are replaced with the letter “z”. The `replaceAll()` method returns a new string with the replacements made, so we need to assign it back to the original variable `str` to update its value.
Javascript replace all string with another replace all / within javascript
To replace all occurrences of a string with another string in JavaScript, you can use the replace() function with a regular expression. In this case, to replace all occurrences of “/” with “-“, you can do:
“`
var str = “some/string/here”;
var newStr = str.replace(/\//g, “-“);
console.log(newStr); // “some-string-here”
“`
Here, we use the regular expression `/\//g` to match all occurrences of “/” in the string, and the “g” flag to replace all of them. We then replace each occurrence with “-“. The resulting string (“some-string-here”) is stored in the variable `newStr`.
YOU CAN ALSO READ: THE ULTIMATE CHECKLIST FOR BLAZOR WEBASSEMBLY
How to Replace all backslashes javascript
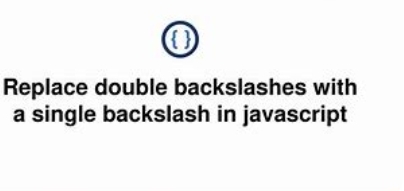
To replace all backslashes in JavaScript, you can use the replace() method with the global regular expression flag (/g) like this:
“`
let str = “This\\ is\\ a\\ backslash\\ string”;
let newStr = str.replace(/\\/g, ‘/’);
console.log(newStr); // Output: “This/ is/ a/ backslash/ string”
“`
In the above code, we first define a string variable “str” that contains backslashes. Then we use the replace() method to replace all backslashes with forward slashes using the regular expression /\\/g. The /g flag ensures that all occurrences of backslashes will be replaced, not just the first one. Finally, we store the new string and replace slashes in a new variable “newStr”.
How to Replace all Brackets javascript
To replace all brackets in a string using JavaScript, you can use the `replace()` method with a regular expression. The regular expression should match all opening and closing brackets and replace them with the desired character or string.
Here’s an example:
“`
let str = “Hello [World]!”;
let newStr = str.replace(/[()\[\]{}]/g, “”);
console.log(newStr); // Output: “Hello World!”
“`
In this example, we’re using the `replace()` method to replace all opening and closing brackets, as well as curly braces, with an empty string. The regular expression `/[()\[\]{}]/g` matches any of the four types of brackets, and the `g` flag ensures that all occurrences within the string are replaced.
You can replace the empty string with any other character or string, depending on your requirements.
Javascript replace all newline with br
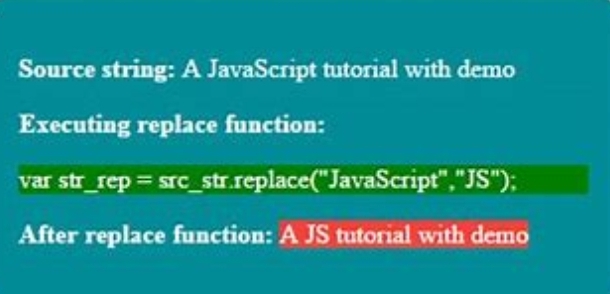
To replace all newline characters with a `<br>` tag in Javascript, you can use the `replace()` method with a regular expression:
“`
var str = “This is a \n multiline \n string.”;
var newStr = str.replace(/\n/g, “<br>”);
console.log(newStr); // “This is a <br> multiline <br> string.”
“`
Here, the regular expression `/\\n/g` matches all newline characters in the string using the `g` flag to replace all occurrences. The second argument to `replace()` is the string to replace each match with. In this case, we replace each newline with the HTML `<br>` tag.
Replace all javascript case insensitive
To replace all occurrences of a string in JavaScript in a case-insensitive manner, you can use a regular expression with the “gi” flags.
For example:
“`
var str = “This is a test string, TEST string”;
var newStr = str.replace(/test/gi, “replaced”);
console.log(newStr); // “This is a replaced string, replaced string”
“`
In this example, the regular expression `/test/gi` matches both “test” and “TEST” in the original string. The “g” flag makes the regular expression global, so it finds all occurrences, and the “i” flag makes it case-insensitive. The `replace()` method then replaces all occurrences with the specified replacement string.
Note that regular expressions can be powerful but also complex. Be sure to test your regular expression thoroughly before using it in production code.
How to replace all javascript commas
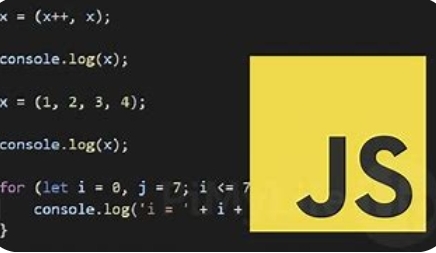
To replace all commas in JavaScript, you can use the String replace() function with a regular expression.
For example, to replace all commas with semicolons in a string variable called str, you can do:
“`js
str = str.replace(/,/g, ‘;’);
“`
The regular expression `/,/g` matches all commas in the string and the `g` flag specifies that all occurrences should be replaced. The semicolon is used as the replacement character in this example but can be replaced with any other character or string.
How to replace all classes javascript
To replace all classes in JavaScript, you can use the `classList` property of an element to manipulate the classes.
1. Get the element(s) which you want to change the class:
“`javascript
const elements = document.querySelectorAll(“.old-class”);
“`
2. Loop through the elements and use the `classList.replace()` method to replace the old class with the new class:
“`javascript
elements.forEach(element => {
element.classList.replace(“old-class”, “new-class”);
});
This will replace all instances of the old class with the new class on the selected elements.
How replace all char javascript
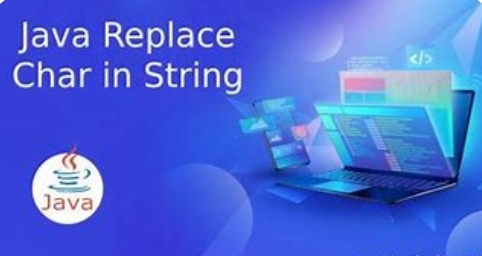
To replace all occurrences of a character in a string in JavaScript, you can use the `replace()` method with a regular expression.
Here’s an example:
“`
let myStr = “Hello World!”;
myStr = myStr.replace(/o/g, “x”);
console.log(myStr);
// Output: Hxllx Wxrld!
“`
In this example, we replace all occurrences of the character “o” in the string “Hello World!” with the character “x”. The regular expression `/o/g` matches all occurrences of the letter “o” in the string and the “g” flag tells the `replace()` method to replace all occurrences, not just the first one.
YOU CAN ALSO READ: THE FRUGAL GUIDE TO LOW CODE DEVELOPMENT (LCD)
How replace all multiple characters javascript
To replace all multiple characters in JavaScript, you can use the `replace()` function with a regular expression. Here is an example:
“`
var string = “helloo”;
string = string.replace(/o+/g, “o”);
console.log(string); // output: “hello”
“`
In this example, the regular expression `/o+/g` matches one or more occurrences of the character “o”. The `g` flag at the end of the regular expression tells `replace()` to match all occurrences of the pattern, not just the first one.
The second argument to `replace()` is the replacement string. In this case, we’re replacing all occurrences of one or more “o” characters with a single “o”.
You can modify the regular expression to match any pattern of multiple characters that you want to replace.
How to replace all special characters javascript
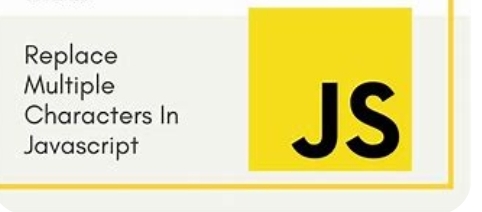
To replace all with javascript special characters using JavaScript, you can use the replace() method with a regular expression that matches all special characters. Here’s a sample code:
“`
function replaceSpecialChars(str) {
return str.replace(/[^\w\s]/gi, ”);
}
// Example usage:
console.log(replaceSpecialChars(“Hello, #world!”)); // Output: “Hello world”
“`
In this code, the regular expression `[^\w\s]` matches any character that is not a word character (letter, number, or underscore) or a whitespace character. The `gi` flags make the regular expression global (to replace all occurrences) and case-insensitive. The replace() method replaces all matched special characters with an empty string, effectively removing them from the string.
Conclusion
the “replace all with javascript” function in JavaScript is an essential tool for developers seeking efficient and concise solutions to string manipulation tasks. Its ability to promptly replace all occurrences of a specified substring simplifies coding workflows and contributes to improved user experiences. By embracing this replace all with javascript function within your JavaScript projects, you’re harnessing the power of a versatile tool that enhances both your codebase and end-user satisfaction.