javascript in array : Are you interested in learning what JavaScript in an array is about?. If so, you are reading the right article. First let’s look at what a JavaScript array is about. JavaScript array is a type of data structure which stores multiple values of the same or different single variable.
What is javascript in an array ?Â

JavaScript is an orderly collection of elements, each of those identified by an index. Arrays used in JavaScript are not limited to specific size; this is because they can grow or shrink dynamically based on the present needs or usage. JavaScript in array are capable of holding different types of data which may include: numbers, strings, objects, and even hold other arrays. Arrays are built in features in JavaScript and are mainly used in web development primarily for storing and manipulating data.
Now that we know what JavaScript in array is, let us now go on to explore other information related to JavaScript in arrays.
YOU CAN ALSO READ: THE USE OF BLAZOR VS JAVASCRIPT IN WEB DEVELOPMENT
Uses of JavaScript in arrayÂ
JavaScript in arrays are very important part of programing language, and they are used in several application. Below are some of the most common used of JavaScript in arrays.
1. Storing data -javascript in arrays are useful for storing data that is related to each other. You can use arrays to store a list of items, like a list of names, phone numbers, or addresses.
2. Sorting data -javascript in array is use in Sorting data is one of the most common applications of arrays. You can use arrays to sort a list of items alphabetically, numerically, or by any other criteria.
3. Searching data – You can use javascript in arrays to search for specific elements in a list. This can be especially useful when you have a large amount of data to sift through, and you need to find a specific item quickly.
4. Filtering data – javascript in arrays can be used to filter data based on specific criteria. For example, you can use arrays to filter out items that don’t meet certain criteria, like a specific color or size.
5. Manipulating data -javascript in arrays allow you to manipulate data quickly and easily. You can add, remove, or change items in an array, and you can perform other operations as well, like merging or splitting arrays.
javascript insert in array at index
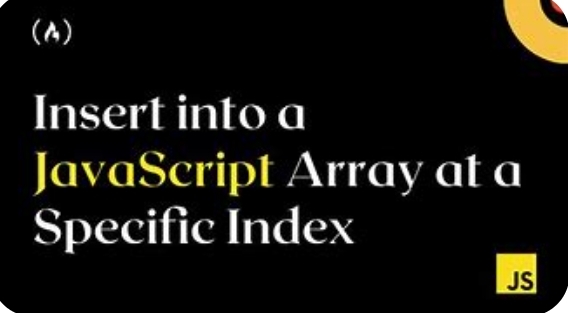
To insert an element into a specific index of an array in JavaScript, you can use the `splice()` method.
Here is an example:
“`javascript
const array = [1, 2, 3, 5];
array.splice(3, 0, 4); // insert 4 at index 3
console.log(array); // output: [1, 2, 3, 4, 5]
“`
The `splice()` method takes three arguments:
1. the index where you want to insert the new element (in this example, it’s `3`)
2. the number of elements you want to delete (in this example, it’s `0` because we’re not deleting anything)
3. the new element you want to insert (in this example, it’s `4`)
After calling `splice()`, the original array will be modified and the new element will be inserted at the specified index.
YOU CAN ALSO READ: THE ULTIMATE CHECKLIST FOR BLAZOR WEBASSEMBLY
javascript find in array and remove?
You can use the JavaScript `indexOf` method to find the position of an element in an array, and then use the `splice` method to remove it. Here’s an example:
“`javascript
let arr = [1, 2, 3, 4, 5];
// Find the index of element 3
let index = arr.indexOf(3);
// If the element exists, remove it
if (index !== -1) {
arr.splice(index, 1);
}
console.log(arr); // [1, 2, 4, 5]
“`
In this example, the `indexOf` method returns the position of element 3 in the array. The `splice` method then removes one element from that position.
Note that if the element you want to remove appears multiple times in the array, this method will only remove the first occurrence. If you want to remove all occurrences, you can use a loop to keep searching for the element until it’s no longer found.
javascript array in an object
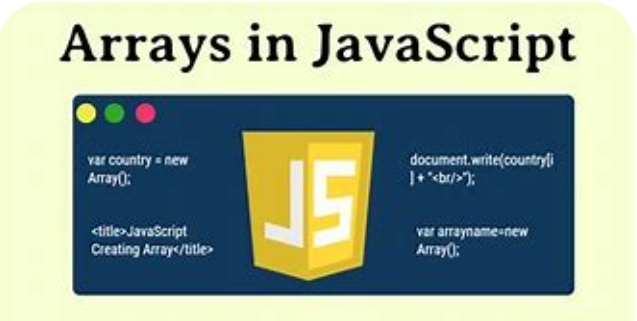
Creating an array within an object in JavaScript can be done in several ways. Here’s an example:
“`
const myObject = {
myArray: [1, 2, 3, 4, 5],
// other properties and methods…
};
“`
In this example, the `myObject` variable is an object with a property called `myArray`. The value of this property is an array containing the numbers 1 through 5.
You can also create an empty array as a property of an object and fill it later:
“`
const myObject = {
myArray: [],
// other properties and methods…
};
myObject.myArray.push(1);
myObject.myArray.push(2);
myObject.myArray.push(3);
“`
In this example, we first create an empty array as the value of the `myArray` property. Then we use the `push()` method to add the numbers 1, 2, and 3 to the array.
javascript array in ascending order
To sort a JavaScript array in ascending order, you can use the `sort()` method. By default, this method sorts an array in alphabetical order, but you can pass in a comparison function to sort the elements in ascending order based on their numeric value.
Here’s an example of sorting an array of numbers in ascending order:
“`javascript
let numbers = [5, 2, 8, 4, 9, 1];
numbers.sort(function(a, b) {
return a – b;
});
console.log(numbers); // [1, 2, 4, 5, 8, 9]
“`
In this example, the comparison function takes two parameters, `a` and `b`, and subtracts `b` from `a`. If the result is negative, it means that `a` is smaller than `b`, so `a` should come before `b` in the sorted array. If the result is positive, it means that `a` is larger than `b`, so `a` should come after `b` in the sorted array. If the result is zero, it means that `a` and `b` are equal, so their original order in the array is preserved.
Alternatively, you can use the arrow function syntax to make the code more concise:
“`javascript
let numbers = [5, 2, 8, 4, 9, 1];
numbers.sort((a, b) => a – b);
console.log(numbers); // [1, 2, 4, 5, 8, 9]
“`
javascript elements in array count
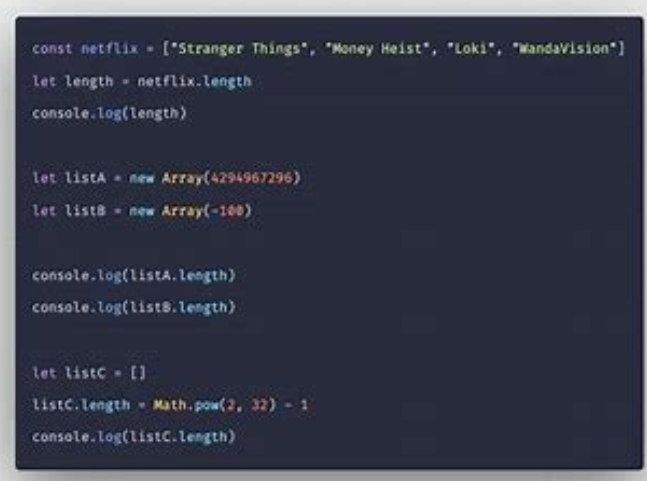
To count the number of elements in a JavaScript array, you can use the `length` property of the array. Here’s an example:
“`javascript
let myArray = [“apple”, “banana”, “cherry”, “date”];
let arrayLength = myArray.length;
console.log(arrayLength); // 4
“`
In this example, we have an array called `myArray` with four elements. We then use the `length` property to get the number of elements in the array, and store it in a variable called `arrayLength`. Finally, we log the `arrayLength` variable to the console.
Note that the `length` property returns the number of elements in the array, not the index of the last element. So if you had an array with five elements, the `length` property would return `5`, not `4`.
javascript find in array by key value
To find an element in an array by a key/value pair in JavaScript, you can use the `find()` method.
Here’s an example:
“`js
const users = [
{ id: 1, name: ‘John’ },
{ id: 2, name: ‘Bob’ },
{ id: 3, name: ‘Jane’ },
];
const targetUser = users.find(user => user.id === 2);
console.log(targetUser); // Output: { id: 2, name: ‘Bob’ }
“`
In the example above, `users` is an array of objects, each containing an `id` and a `name`. We want to find the user whose `id` is `2`, so we use the `find()` method and pass in a function that checks if the `id` of each user matches `2`. Once the function finds a matching user, it returns that user object. Finally, we log the `targetUser` to the console.
javascript find in array by property value

To find an object in an array by a specific property value in JavaScript, you can use the `find()` method.
Here’s an example:
“`
let myArray = [
{name: ‘John’, age: 25},
{name: ‘Alice’, age: 30},
{name: ‘Bob’, age: 35},
];
let result = myArray.find(item => item.name === ‘Alice’);
console.log(result); //{name: ‘Alice’, age: 30}
“`
In this example, we are using the `find()` method to search through the `myArray` for an object whose `name` property is equal to “Alice”. The `find()` method will return the first object it finds that matches the condition.
Note that if no object is found with the property value, the `find()` method will return `undefined`.
javascript object in array access
JavaScript allows you to access objects in an array by using their index position within the array. For example:
“`
const myArray = [
{ name: ‘John’, age: 28 },
{ name: ‘Sarah’, age: 30 },
{ name: ‘Peter’, age: 25 }
];
console.log(myArray[0]); // output: { name: ‘John’, age: 28 }
console.log(myArray[1].name); // output: Sarah
“`
In the above example, we have an array of three objects, each containing a `name` and `age` property. To access the first object in the array, we simply use the index position `[0]`. To access the name property of the second object in the array, we use the index position `[1]` followed by the property name `.name`.
You can also loop through an array of objects and access each object’s properties using a `for` loop or a `forEach()` method. For example:
“`
myArray.forEach(item => {
console.log(item.name);
});
“`
This will log the names of each object in the array to the console.
YOU CAN ALSO READ : THE FRUGAL GUIDE TO LOW CODE DEVELOPMENT (LCD).
javascript array api
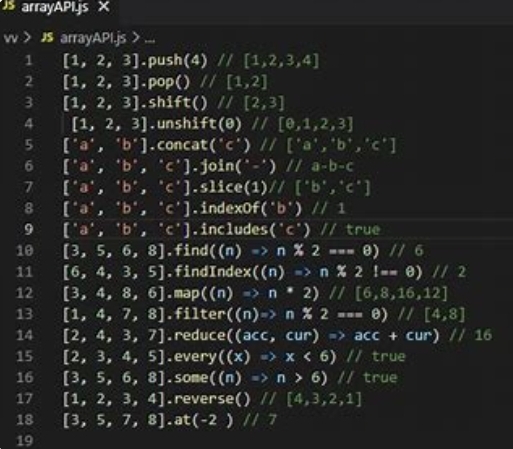
JavaScript provides a number of built-in Array APIs (Application Programming Interfaces), which allow developers to work with arrays easily and efficiently. Here are some of the most commonly used Array APIs in JavaScript:
1. push() – Adds one or more elements to the end of an array.
2. pop() – Removes the last element from an array.
3. unshift() – Adds one or more elements to the beginning of an array.
4. shift() – Removes the first element from an array.
5. indexOf() – Returns the index of the first occurrence of a specified element in an array.
6. slice() – Returns a new array that is a shallow copy of a portion of the original array.
7. splice() – Adds or removes elements from an array at a specified position.
8. concat() – Returns a new array that is a combination of two or more arrays.
9. forEach() – Calls a function once for each element in an array.
10. map() – Creates a new array with the results of calling a function for each element in an array.
11. filter() – Creates a new array with all elements that pass the test implemented by the provided function.
12. reduce() – Applies a function to each element in an array in order to reduce the array to a single value.
13. reverse() – Reverses the order of the elements in an array.
14. sort() – Sorts the elements of an array in place.
These Array APIs make it easier for developers to perform various operations on arrays, such as adding or removing elements, manipulating the contents of the array, or iterating through the array to perform some action on each element.
Conclusion
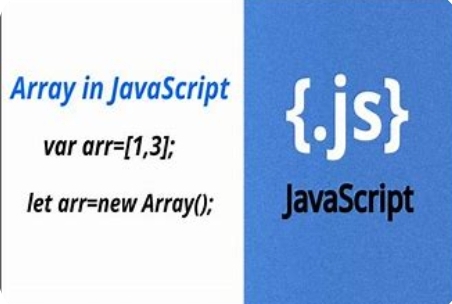
JavaScriptin in array are a fundamental data structure that allows developers to organize and store multiple elements of data in a single variable. They provide a powerful and flexible way to manipulate data and are used extensively in web development for various applications, including dynamic content creation, data sorting, and filtering. There are numerous built-in methods available to manipulate arrays, making them easy to work with and use. The versatility and utility of arrays make them an essential tool in the JavaScript toolkit for any programmer.
Arrays play a fundamental role in programming with javascript in array. They are most times used to store and organize collections of information in a single variable. In javascript, an array can hold elements of different data types such as strings, numbers, and even other arrays. Manipulating data with javascript in array is a common task, and arrays provide various methods that facilitate this process.
One of the essential aspects of javascript in array is accessing its elements. Elements in an array are indexed starting from zero. This means that the first element is at index 0, the second at index 1, and so on. You can use these indices to retrieve or modify the elements stored in the javascript in array.
Adding and removing elements in javascript in array can be achieved using various methods. The “push” method is used to add elements to the end of the array, while the “pop” method removes the last element. Similarly, the “unshift” method adds elements to the beginning, and the “shift” method removes the first element of the javascript in array.